Text to Video Endpoint
Overview
Text to Video endpoint generates and returns a video based on a text description.
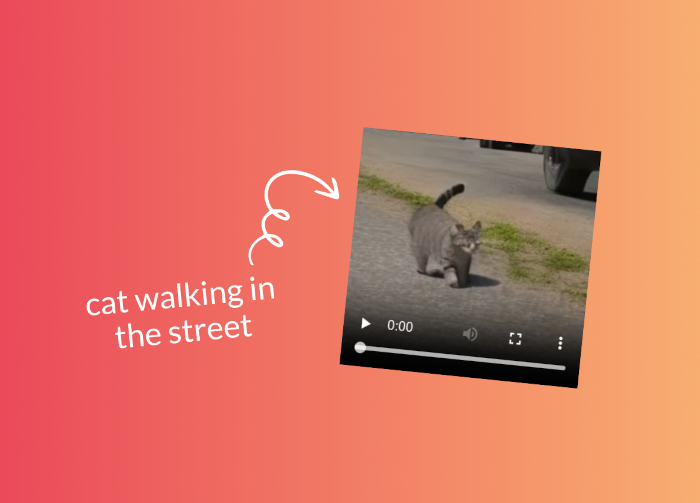
Request
--request POST 'https://stablediffusionapi.com/api/v5/text2video' \
Make a POST
request to https://stablediffusionapi.com/api/v5/text2video endpoint and pass the required parameters in the request body.
Body Attributes
Parameter | Description |
---|---|
key | Your API Key used for request authorization. |
prompt | Text prompt with description of the things you want in the video to be generated. |
negative_prompt | Items you don't want in the video. |
scheduler | Use it to set a scheduler for video creation. |
seconds | Duration of the video in seconds. |
Schedulers
This endpoint also supports schedulers. Use the "scheduler" parameter in the request body to set a specific scheduler to be used from the list below:
- DDPMScheduler
- DDIMScheduler
- PNDMScheduler
- LMSDiscreteScheduler
- EulerDiscreteScheduler
- EulerAncestralDiscreteScheduler
- DPMSolverMultistepScheduler
- HeunDiscreteScheduler
- KDPM2DiscreteScheduler
- DPMSolverSinglestepScheduler
- KDPM2AncestralDiscreteScheduler
- UniPCMultistepScheduler
- DDIMInverseScheduler
- DEISMultistepScheduler
- IPNDMScheduler
- KarrasVeScheduler
- ScoreSdeVeScheduler
- LCMScheduler
Example
Body
Body
{
"key": "",
"prompt": "man walking on the road, ultra HD video",
"negative_prompt": "Low Quality",
"scheduler": "UniPCMultistepScheduler",
"seconds": 3
}
Request
- JS
- PHP
- NODE
- PYTHON
- JAVA
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"key": "",
"prompt": "man walking on the road, ultra HD video",
"negative_prompt": "Low Quality",
"scheduler": "UniPCMultistepScheduler",
"seconds": 3
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://stablediffusionapi.com/api/v5/text2video", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$payload = [
"key" => "",
"prompt" => "man walking on the road, ultra HD video",
"negative_prompt" => "Low Quality",
"scheduler" => "UniPCMultistepScheduler",
"seconds" => 3
];
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://stablediffusionapi.com/api/v5/text2video',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => json_encode($payload),
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://stablediffusionapi.com/api/v5/text2video',
'headers': {
'Content-Type': 'application/json'
},
body: JSON.stringify({
"key": "",
"prompt": "man walking on the road, ultra HD video",
"negative_prompt": "Low Quality",
"scheduler": "UniPCMultistepScheduler",
"seconds": 3
})
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
import requests
import json
url = "https://stablediffusionapi.com/api/v5/text2video"
payload = json.dumps({
"key": "",
"prompt": "man walking on the road, ultra HD video",
"negative_prompt": "Low Quality",
"scheduler": "UniPCMultistepScheduler",
"seconds": 3
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n \"key\": \"\",\n \"prompt\" : \"man walking on the road, ultra HD video\",\n \"negative_prompt\" : \"Low Quality\",\n \"scheduler\" : \"UniPCMultistepScheduler\",\n \"seconds\" : 3\n}");
Request request = new Request.Builder()
.url("https://stablediffusionapi.com/api/v5/text2video")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.build();
Response response = client.newCall(request).execute();
Response
Example Response
{
"status": "success",
"generationTime": 9.029465913772583,
"id": 14758180,
"output": [
"https://pub-8b49af329fae499aa563997f5d4068a4.r2.dev/generations/ee3e20f4-adf7-4cb2-9733-3c3b6bae8813.mp4"
]
}