Fetch Queued Images Endpoint
Overview
Stable Diffusion V3 APIs Fetch Queued Images API fetches queued images from stable diffusion.
Usually more complex image generation requests take more time for processing. Such requests are being queued for processing and the output images are retrievable after some time.
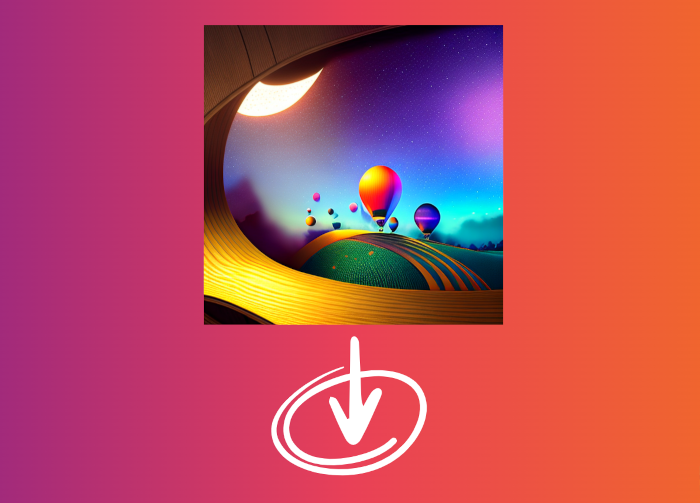
Request
--request POST 'https://stablediffusionapi.com/api/v3/fetch/{id}' \
Send a POST
request to https://stablediffusionapi.com/api/v3/fetch/{id} endpoint to return the corresponding queued images. Where {id}
is the ID returned together with the image URL in the response upon its generation.
This endpoint does not generate new images, it returns already generated/queued images.
Attributes
Parameter | Description |
---|---|
key | Your API Key used for request authorization |
Example
Body
Body Raw
{
"key": ""
}
Request
- JS
- PHP
- NODE
- PYTHON
- JAVA
var myHeaders = new Headers();
myHeaders.append("Content-Type", "application/json");
var raw = JSON.stringify({
"key": ""
});
var requestOptions = {
method: 'POST',
headers: myHeaders,
body: raw,
redirect: 'follow'
};
fetch("https://stablediffusionapi.com/api/v3/fetch/12202888", requestOptions)
.then(response => response.text())
.then(result => console.log(result))
.catch(error => console.log('error', error));
<?php
$payload = [
"key" => ""
];
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://stablediffusionapi.com/api/v3/fetch/12202888',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'POST',
CURLOPT_POSTFIELDS => json_encode($payload),
CURLOPT_HTTPHEADER => array(
'Content-Type: application/json'
),
));
$response = curl_exec($curl);
curl_close($curl);
echo $response;
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://stablediffusionapi.com/api/v3/fetch/12202888',
'headers': {
'Content-Type': 'application/json'
},
body: JSON.stringify({
"key": ""
})
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
import requests
import json
url = "https://stablediffusionapi.com/api/v3/fetch/12202888"
payload = json.dumps({
"key": ""
})
headers = {
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data=payload)
print(response.text)
OkHttpClient client = new OkHttpClient().newBuilder()
.build();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\n \"key\": \"\"\n}");
Request request = new Request.Builder()
.url("https://stablediffusionapi.com/api/v3/fetch/12202888")
.method("POST", body)
.addHeader("Content-Type", "application/json")
.build();
Response response = client.newCall(request).execute();
Response
Example Response
{
"status": "success",
"id": 12202888,
"output": [
"https://pub-8b49af329fae499aa563997f5d4068a4.r2.dev/generations/e5cd86d3-7305-47fc-82c1-7d1a3b130fa4-0.png"
]
}